SwiftUI TextField 사용하기
이번에는 SwiftUI에서 TextField를 어떻게 사용하는지에 대해 알아보겠습니다.
Text 읽어오기
- TextField는 Text의 입력을 읽어오기 위한 component 입니다.
- 우선, TextField를 사용하기 위해서는
TextField
명령어를 사용하면 되는데요. TextField
의 인자 값으로 @State로 선언된 변수를 넣어주면, 이 변수에 텍스트가 입력됩니다.
struct ContentView : View {
@State var name: String = ""
var body: some View {
VStack {
TextField("Enter your name", text: $name)
.padding()
.background(Color(uiColor: .secondarySystemBackground))
Text("Hello \(name)")
}
}
}
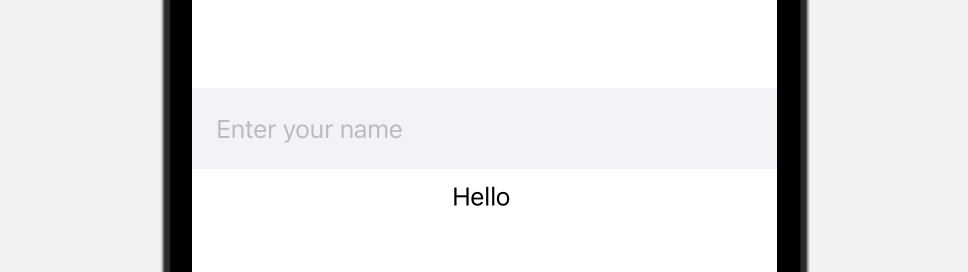
TextField placeholder
- 사실, placeholder은 위에서도 사용 했는데요.
- Textfield의 첫 번째 인자 값으로 placeholder 텍스트가 입력됩니다.
- 예를들어, 이와 같은 코드는 기본으로 Enter your email을 띄워주고, 입력되는 값을 emailAddress 변수에 담아줍니다.
struct ContentView : View {
@State var emailAddress: String = ""
var body: some View {
VStack {
TextField("Enter your email", text: $emailAddress)
.padding()
.background(Color(uiColor: .secondarySystemBackground))
Text("Email \(emailAddress)")
}
}
}
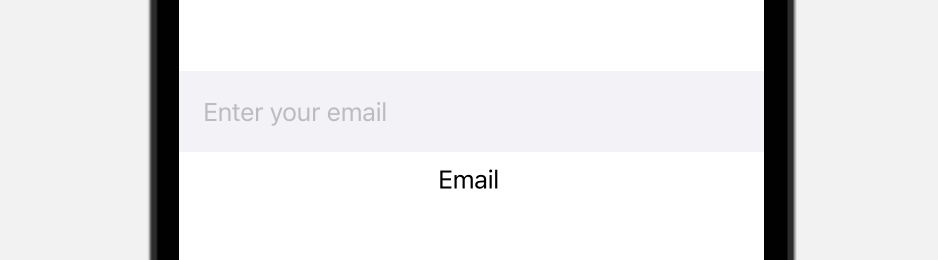
TextField 스타일
- TextField의 스타일을 변경하기 위해서는 textFieldStyle을 사용하면 됩니다.
- 특히, 텍스트필드에 테두리 스타일을 주기 위해서는 roundedBorder을 사용하면 됩니다.
struct ContentView : View {
@State var emailAddress: String = ""
var body: some View {
VStack {
TextField("Enter your email", text: $emailAddress)
.padding()
.background(Color(uiColor: .secondarySystemBackground))
.textFieldStyle(.roundedBorder)
Text("Email \(emailAddress)")
}
}
}
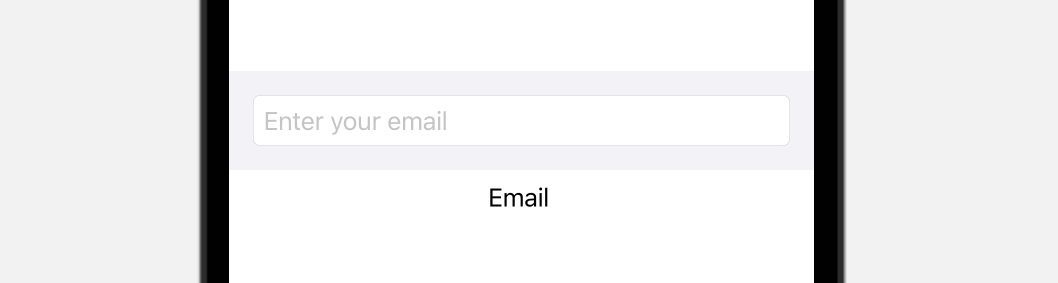
Secure TextField
- Secure Textfield는 입력되는 텍스트의 값을 보호해주기 때문에, 암호와 같은 텍스트를 입력 받을 때 사용됩니다.
- 기본적으로, TextField와 사용 법이 비슷합니다.
- SecureField 명령어를 입력하고 첫 인자 값으로 placeholder 텍스트를, 두 번째 입력 값으로 입력 받은 텍스트를 담을 변수를 넣어주면 됩니다.
struct ContentView : View {
@State var password: String = ""
var body: some View {
VStack {
SecureField("Enter a password", text: $password)
.padding()
.background(Color(uiColor: .secondarySystemBackground))
.textFieldStyle(.roundedBorder)
Text("You entered: \(password)")
}
}
}
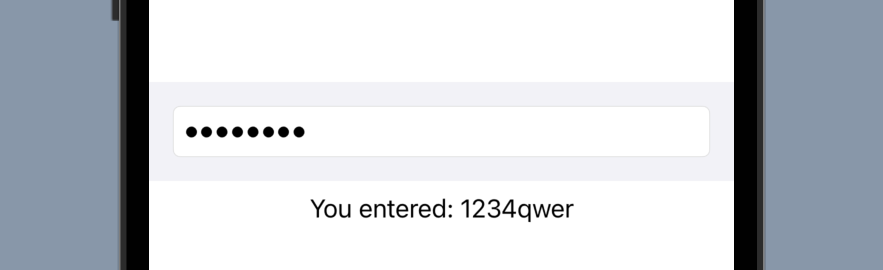
TextEditor
- 여러 줄의 텍스트를 입력 받기 위해서는 TextEditor을 사용하면 됩니다.
struct ContentView : View {
@State var text: String = ""
var body: some View {
TextEditor(text: $text)
.cornerRadius(15)
.padding()
.background(.blue)
}
}
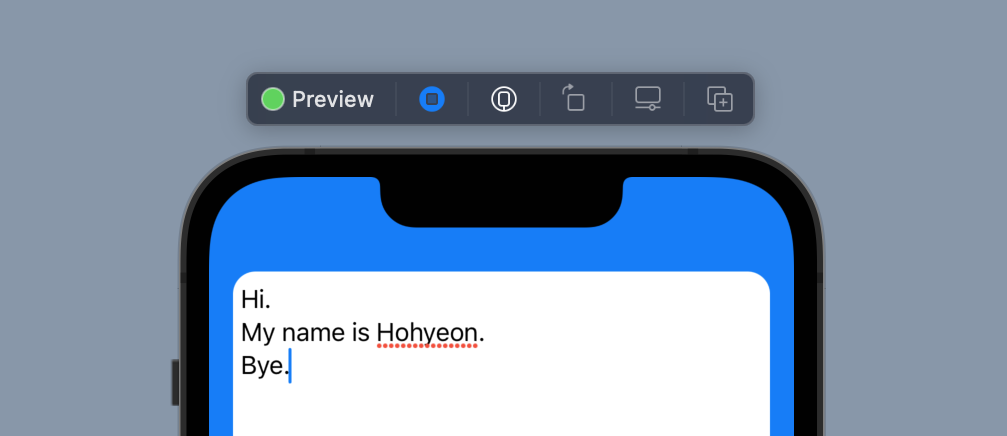
마무리
- 이렇게 해서 SwiftUI에서는 TextField를 어떻게 사용하는지 알아봤습니다.